POST IS WORK IN PROGRESS
Events are one of three ways to provide data from an OPC UA server. Important to note, it is a completely different mechanism than reading or subscribing to OPC UA variables.
When to use OPC UA Events?
- It is one possible way to provide several consistent values in one transaction.
- Events allow certain kind of flexibility. Events can be extended with additional values in a compatible way via a new inherited type. And the client can choose which values it wants to get. This is done with a so called event filter.
- Events are a good way to model and transport other information than raw data values. Events should be used to indicate that certain things “happen.” For example state changes like a process has started or a process is completed.
- Events can be used to inform about changes in the OPC UA address space. For example there is the BaseModelChangeEvent.
- Finally Events are the foundation for the alarms and conditions system.
How to subscribe to Events?
- Event instances are not visible in the address space, but the EventType definitions are. The EventType defines the content of an event like a template.
- An object node and an event filter is needed to subscribe to events. Using the event filter, the subscription can be limited to certain EventTypes and certain attributes.
- Every OPC UA server has to provide all it’s events via the predefined Server-Object node. Additionally it can provide the possibility to register for events on other object nodes.
- An object node can be used for event subscription, when the attribute “EventNotifier” (https://reference.opcfoundation.org/v104/Core/docs/Part3/5.5.1/) is set to 1. That attribute is defined in the object node class, so it is an attribute of every object.
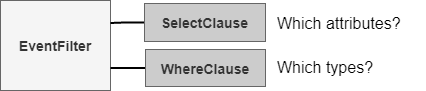
Example event hierarchy:
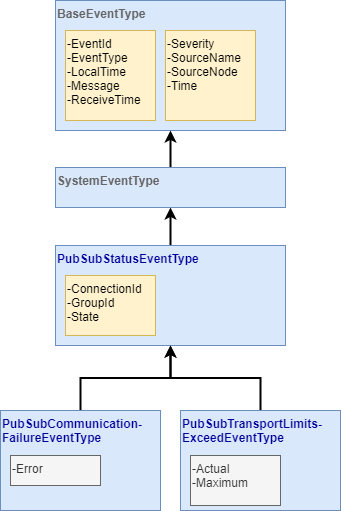
Code example with Python:
In python there is a great high level api, which allows to program OPC UA stuff with very few lines of code. In python adding a filter is optional. If no filter is passed, it is auto created. The example uses the sampling interval 0 and the PubSubStatusEventType.
sub = await client.create_subscription(0, callback) h = await sub.subscribe_events(client.nodes.server, pubSubStatusEventType)
Code example with .NET:
The .NET library from OPCFoundation is more low level and needs more code. In the examples a helper class “FilterDeclaration” is used to auto create the event filter.
// the filter to use. m_filter = new FilterDeclaration(pubSubStatusEventType, null); // create a monitored item based on the current filter settings. m_monitoredItem = new MonitoredItem(); m_monitoredItem.StartNodeId = Opc.Ua.ObjectIds.Server; m_monitoredItem.AttributeId = Attributes.EventNotifier; m_monitoredItem.SamplingInterval = 0; m_monitoredItem.QueueSize = 1000; m_monitoredItem.DiscardOldest = true; m_monitoredItem.Filter = m_filter.GetFilter(); m_subscription.AddItem(m_monitoredItem);
How is the filter auto created?
Use UaExport to view Events
Custom Event Filter
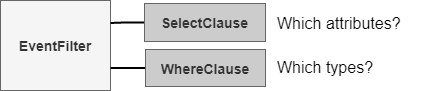
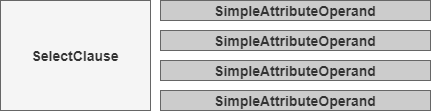
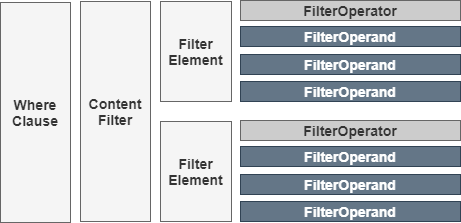
Two ways to implement the ContenFilter for EvenTypes:
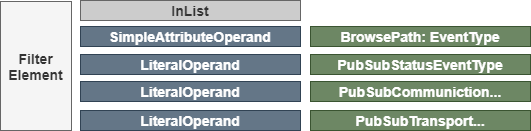
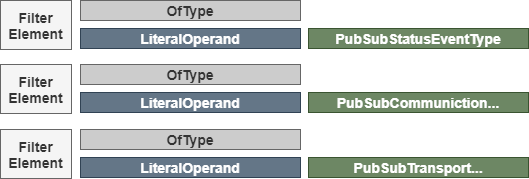
For ConditionEvents a special entry is necessary in the SelectClause to get the ConditionId (NodeId). See bottom of ConditionType Model.